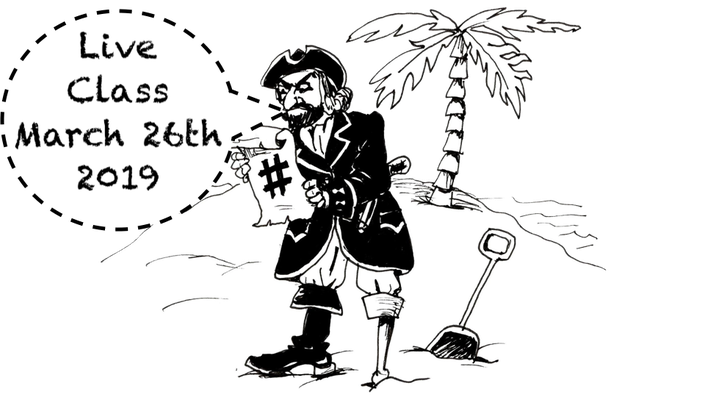
Data Structures in Java Live Class 2019-03-26
A thorough look at the collection classes in java.util.** in Java
Our live classes are different every time we run them. We do not promise to stick to the schedule, but will move as the students direct us. Some live classes are fantastic. Others just great. Your call.
Students Registered (50/50) - Sold Out
Please Email Us so we can inform you when the next class is scheduled.
This is a live virtual class running on March 26th, 2019 from 07:00-11:00 PDT, 10:00-14:00 EDT, 14:00-18:00 UTC, 19:30-23:30 IST.
Anyone registered will get access to the recording. In addition, they get access to the previous Data Structures Live Class Recording from the 21st February 2019, the Data Structures in Java and Build Your Own Circular Array List.
Java programmers typically use only very few collection classes for all of their work. Often they chose inappropriate ones. In this course, we will look at what each collection costs and when you should use which one. Some of the questions will we answer include: Which is the best Collection to use in Java? When do you need to employ ConcurrentSkipListSet? What is the computational time complexity of WeakHashMap?
What you'll learn-and how you can apply it
- Java has an overwhelming number of interfaces and classes in the java.util.** packages. In this course you will learn what they are and when to use which. Is ArrayList better than LinkedList? What is the difference in space complexity between the two classes?
- Choosing the correct collection can make our code more succinct. For example, the new List.of() syntax in Java 9 is better at creating immutable lists.
- By looking at hashing closely, we can learn how to write hashing functions that produce a 3x performance improvement for HashMap and ConcurrentHashMap.
- We learn when to use which concurrent classes and which to avoid.
This training course is for you because...
- Junior and intermediate Java programmers wanting to understand collections better.
- Advanced programmers who want to acquire additional skills.
Prerequisites
- Attendees should be familiar with basic Java code, but a CS degree is not assumed
- Should know at least Java 6. We are covering all relevant collection classes up until Java 11. Knowledge of streams and lambdas not necessary.
Recommended Follow-up
Sign up to The Java Specialists’ Newsletter for lots of tips on the latest Java developments: https://www.javaspecialists.eu/archive

"I enjoyed the data structures course, appreciate it not being a rehash of the self study course :)"
This course is closed for enrollment.
Schedule
The timeframes are only estimates and may vary according to how the class is progressing
0 - Welcome
(5m @07h00-07h05 5m)
- Everything is in the recording
- Heinz and his IDE
- Quizzes
- Answer in chat
- Questions
1 - Introduction to Collections in Java
(10m @07h05-07h15 15m)
- Computational Time and Space Complexity
- O(1), O(n), O(n2), O(log n), O(n * log n)
- Arrays
- Primitive vs object arrays
- Memory usage and layout
- Multi-dimensional arrays
Quiz
(5m @07h15-07h20 20m)
2 - Lists
(30m @07h20-07h50 50m)
- Lists
- Arrays.asList()
- Quick look at the List methods
- Optional methods
- asList() vs List.of()
- RandomAccess
- ArrayList
- Adding four seasons
- indexOf() and contains()
- size() vs elementData.length in debugger
- removeIf()
- Iteration
- Enumeration bugs
- Fail fast collection
- forEach()
- CopyOnWriteArrayList
- Safe iteration
- LinkedList
- Node memory usage
- Accessing middle of list
Quiz
(5m @07h50-07h55 55m)
7 Minute Break
(7m @07h55-08h02)
3 - Sorting
(25m @08h02-08h27 25m)
- Sorting lists
- Sorting list of Strings
- Sorting custom classes like Student
- Comparing ints and longs
- Writing Comparators as anonymous classes
- Comparators with extractor functions
- Type witnesses
- Declared lambda parameters
- Method references
- Sorting performance ArrayList vs LinkedList
- Parallel sorting of ArrayList
Quiz
(5m @08h27-08h32 30m)
4 - Sets
(15m @08h32-08h47 45m)
- Sets
- Set.of()
- union with addAll()
- intersection with retainAll() or stream/filter
- TreeSet
- Sorted by natural order
- Red-black tree
- Unbalanced tree O(n) vs O(log n)
- Counting maximum tree depth
- ConcurrentSkipListSet
- Thread-safe sorted set
- CopyOnWriteArraySet
- For very small sets
Quiz
(5m @08h47-08h52 50m)
7 Minute Break
(7m @08h52-08h59)
5 - Hashing
(15m @08h59-09h14 15m)
- Hashing
- Writing very basic hashtable
- Clashes and distribution
- % vs &
- HashSet
- hashCode() vs identityHashCode()
- Pixel and good hash code
- Bucket collisions
- Making keys implement Comparable
- ConcurrentHashMap.newKeySet()
Quiz
(5m @09h14-09h19 20m)
6 - Maps Part 1
(30m @09h19-09h49 50m)
- Maps
- one-to-one dictionary
- HashMap
- History of hashing 1.2, 1.4, 1.8+
- Building a hash code with bit shifting Person(name,day,month,year)
- Cached hash code in Strings
- MapClashInspector
- Creating maps of numbers
- computeIfAbsent() for List of values
7 Minute Break
(7m @09h49-09h56)

"Today I attended a live webinar with Dr. Heinz Kabutz. Even though it was a 4 hour class which started quite early at my time zone, and about #datastructures in #Java - a topic with which I'm very familiar - I found it to be fun and informative.
... the interaction and ability to ask questions in real time was great (I may have asked too many questions lol).
I highly recommend his courses and webinars to anyone who's seeking to improve their #programming."
- Igal Sapir, Software Architect, Tomcat Committer, Lucee Committer
6 - Maps Part 2
(15m @09h56-10h11 15m)
- ConcurrentHashMap
- Always use
- Compound operations review
- TreeMap
- hashCode(), equals() and compareTo()
- Own Comparator
- ConcurrentSkipListMap
- Parallel put into TreeMap vs ConcurrentSkipListMap
- LinkedHashMap and LinkedHashSet
- Highly Specialized Collections: EnumSet, EnumMap, IdentityHashMap, Properties, WeakHashMap
Quiz
(5m @10h11-10h16 20m)
7 - Queues and Deques
(10m @10h16-10h26 30m)
- Queues and Deques
- Not always FIFO
- ConcurrentLinkedQueue and ConcurrentLinkedDeque
- General purpose MPMC queues
- size() is O(n)
- ArrayDeque
- Grows, does not shrink
- BlockingQueues
- LinkedBlockingQueue and LinkedBlockingDeque
- Lock splitting
- ArrayBlockingQueue
- Compact array structure
- Highly specialized queues: DelayQueue, SynchronousQueue, LinkedTransferQueue, PriorityQueue and PriorityBlockingQueue
Quiz
(5m @10h26-10h31 50m)
4 Minute Break
(4m @10h31-10h35)
8 - Collection Facades
(10m @10h35-10h45 10m)
- java.util.Collections
- java.util.Arrays
Quiz
(5m @10h45-10h50 15m)
9 - Wrap-up
(10m @10h50-11h00 25m)
Further questions
"For me it was a very interesting revisit to data structures. It is very educational to follow along and hear your comments as you code. Interesting to learn about alternate syntaxes etc , not always obvious to me :-), but that's the objective of the webinars to show new or advanced ways of coding."
Course Curriculum
Courses Included with Purchase
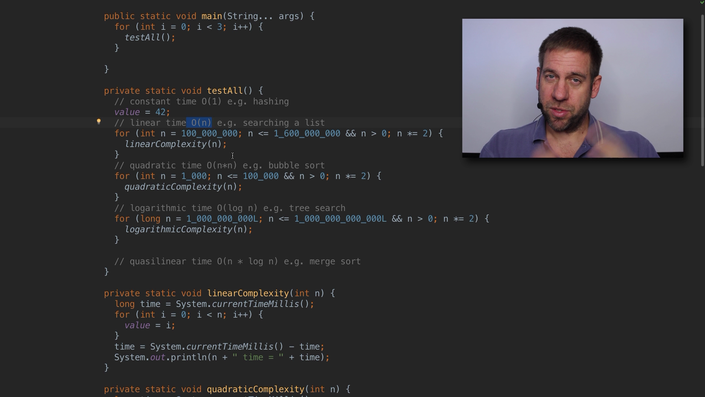

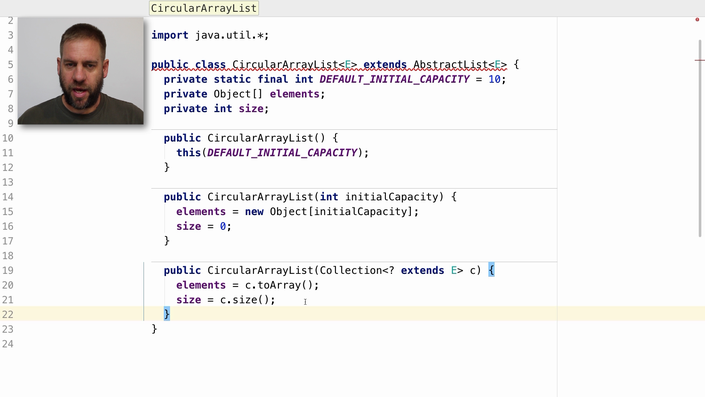

Original Price:
This course is closed for enrollment.
Your Instructor

Heinz Kabutz is the author of The Java Specialists’ Newsletter, a publication enjoyed by tens of thousands of Java experts in over 150 countries. He has been a Java Champion since 2005.