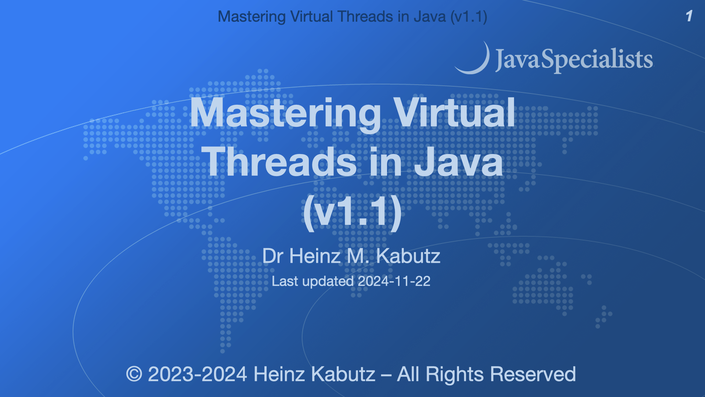
Mastering Virtual Threads in Java (v1.1)
Learn how virtual threads work in Java, including Structured Concurrency, Scoped Values, and how to find and diagnose issues such as deadlocks.
This advanced course dives deep into the world of Virtual Threads introduced in Project Loom, part of Java's modern concurrency paradigm. Designed for experienced Java developers, it explores lightweight threading models for scalable, concurrent programming. Participants will learn to implement virtual threads for optimized resource utilization, replace legacy constructs like ThreadLocal with Scoped Values, and leverage Structured Concurrency for clear, maintainable code.
Through hands-on exercises, real-world use cases, and best practices, this course emphasizes problem-solving and debugging techniques while navigating challenges like thread pinning and deadlocks. Master the tools and techniques necessary to harness the full potential of Virtual Threads, from understanding JVM-level intricacies to refactoring legacy codebases.
Java Specialists Certificates
This course is required to obtain the following Java Specialists Certificates:
Your Instructor

Heinz Kabutz is the author of The Java Specialists’ Newsletter, a publication enjoyed by tens of thousands of Java experts in over 150 countries. He has been a Java Champion since 2005.
Course Curriculum
-
Start1. Introduction to Virtual Threads (7:38)
-
Start1.1. Exercise 1.1 walkthrough (1:21)
-
Start1.2. Daemon Threads (1:19)
-
Start1.2.1. Exercise 1.2. Walkthrough (1:10)
-
Start1.3. Virtual Thread Attributes (3:02)
-
Start1.3.1. Exercise Walkthrough 1.3 (3:55)
-
Start1.4. Carrier Threads (5:49)
-
Start1.4.1. Exercise Walkthrough 1.4 (2:42)
-
Start1.5. Thread Builders (10:09)
-
Start1.6. Timers (6:15)
-
Start1.6.1. Exercise Walkthrough 1.5. (7:11)
-
Start1.7. java.lang.ref.Cleaner (2:34)
-
Start1.8. GC Roots (5:49)
-
Start1.8.1. Exercise 1.6. Walkthrough (6:26)
-
Start1.9. Parallel vs Concurrent Computing (4:21)
-
Start1.10.1. Best Deal Search (29:40)
-
Start1.10.2. Using CompletableFuture (6:27)
-
Start1.10.3. Using Virtual Threads (2:12)
-
Start1.11. States of Virtual vs Platform Threads (4:48)
-
Start1.11.1. java.lang.Thread States (2:14)
-
Start1.11.2. Virtual Thread States (16:10)
-
Start1.11.3. Exercise 1.7 Walkthrough (24:23)
-
Start2. Changes in java.util.concurrent (1:07)
-
Start2.1. AutoCloseable ExecutorService (2:55)
-
Start2.2. Using ExecutorService non-structured (2:07)
-
Start2.3. Using ExecutorService With Deadline (2:28)
-
Start2.4. ManagedBlocker (1:26)
-
Start2.5. Pooling vs Virtual Threads (0:19)
-
Start2.6. Locking in Virtual Threads (1:10)
-
Start2.6.1. Object.wait() in Java 21 (0:29)
-
Start2.6.2. Replace with ReentrantLock and Condition (1:28)
-
Start2.6.3. Blocking while synchronized - pinned (0:57)
-
Start2.6.4. Converting synchronized to ReentrantLock (2:13)
-
Start2.6.5. Java 24 - JEP 491 (3:54)
-
Start2.7. Exercises description (1:21)
-
Start2.7.1. Exercise 2.1. Walkthrough (7:13)
-
Start2.7.1. Exercise 2.2. Walkthrough (16:30)